前回、基本的なところをお試ししてみましたが、
もう少しなんかやらせてみたいので、数あてゲームをやらせてみます。
ランダムな数字を、ヒント(High or Low)を基に当てる超楽しいゲームね。。。
実用性は皆無ですが、条件分岐、ループ、演算と、一通りのことを試すのにはいいかなと。
HTMLはこんな感じにしました。
まぁ、素直なコードかと。。。
問題のNightmare部ですが、ループや条件分岐を使うので、Promise/thenではきついと思われます。
ので、Generatorとvoを使用します。
|
|
こちらを激しく参考にさせてもらいました
んで、できたコードがこちら。
Generatorの使い方がミソですな。
今回function/yieldの概念を初めて使いましたが、<a href=“https://developer.mozilla.org/ja/docs/Web/JavaScript/Reference/Statements/function” rel=“noopener” target=”_blank”>この辺読んでも意味が分かりません。
要はyield使うときは「function*」にしとけってことでしょうか?
今までコールバックとかPromiseとかで独特な記述になってた所が、素直に書けるようになったようです。
印象としてはC#のasync/awaitみたいな感じでしょうかね。
JSエンジンによってかなり実装が違うようです。ブラウザ向けのスクリプトじゃまだ使えなさそう。
実行するとこんな感じ
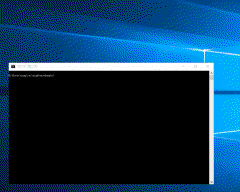
Nightmare使えそうです。
MoneyForwardが非対応なサービスのデータを、自動で取り込めるようにしたいなぁ。
さしあたってAEONのネットスーパー。対応してくんないかな。